Exercise 1 – calling a Hugging Face Transformer API
I chose this exercise because it is free, it will introduce you to a lot of integral tools and concepts behind APIs, and Hugging Face APIs are quite popular, so you will get hands-on experience with those. The API that we will be using specifically is a transformer that turns a written prompt into an image. It’s a great API to learn and find out how APIs in general work. For this lesson, I am using a Google Colab notebook, which is a Jupyter Notebook hosted by Google. It’s pretty useful when you want to recreate runtimes for certain sections of code. It’s like having your own little test section that you can divide into even smaller sections if you want to. Let’s make a notebook to further explore our Hugging Face API:
- To open a Colab notebook, you can go to colab.research.google.com and create a new notebook. The end result should be something like this:
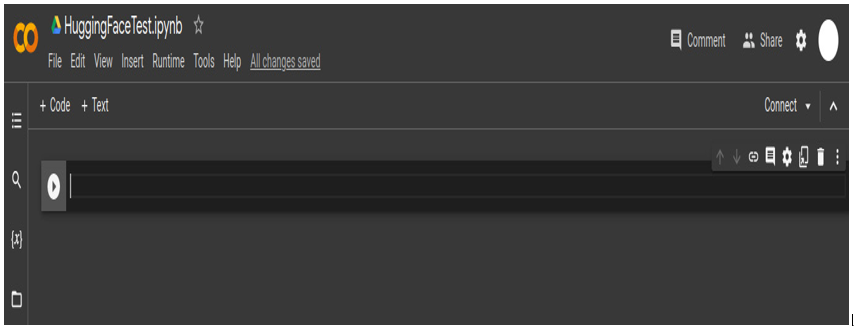
Figure 3.1 – Initial notebook created with Google Colab
- The first thing we need to do is install the correct libraries. These libraries contain the functions and modules with which we call our APIs. You can install them directly in the notebook if you’d like. We are going to install the huggingface_hub and transformers[agents] libraries. Here is the command for this:
!pip install huggingface_hub transformers[agents]
When you put this command in the cell and press play, it will install the libraries in your runtime:
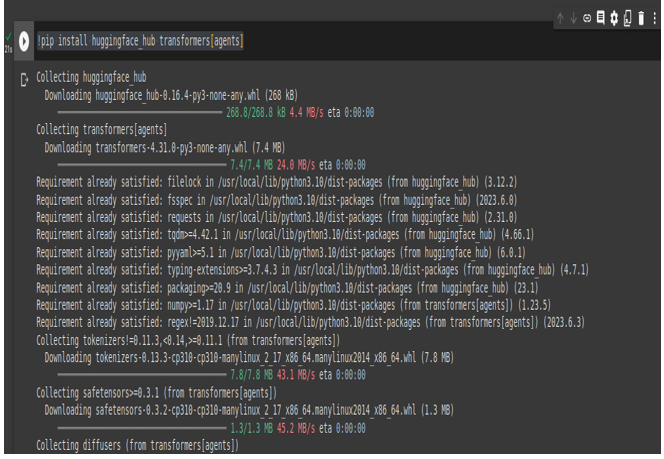
Figure 3.2 – Installing required libraries
- The next thing that you need to do is log in to huggingface_hub using an API key.
This is where the concept of the API key comes from. An API key is like a login but for your software. Most companies only allow full access to their APIs through the purchase of an API key. A lot of open source projects such as Hugging Face have API keys to promote and track user interaction and sometimes upgrade their users to a premium version if they want. - To get a Hugging Face API key, you must first go to the huggingface.co web page and sign up or log in if you’ve already signed up. After doing that, go to your profile and then to the Settings tab and into the Access Tokens tab from there. You can generate an access token for use there:
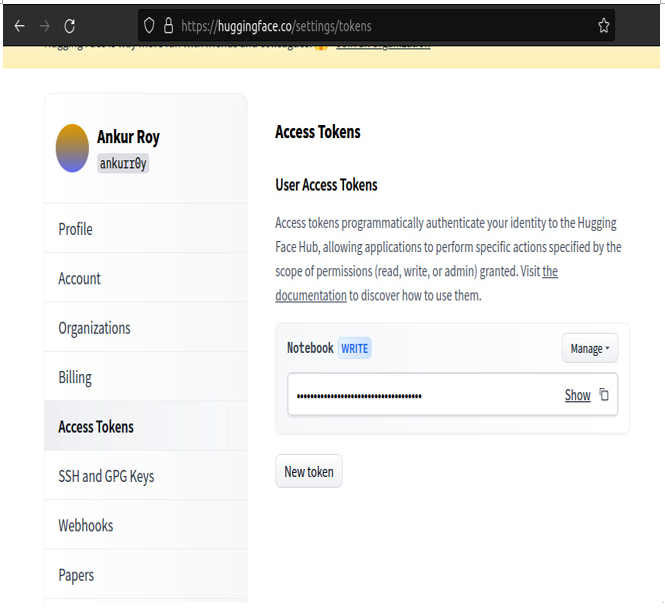
Figure 3.3 – Generating an access token for the Hugging Face API
- You can copy this token for use in your next section of code. Here, you import the Hugging Face login module for the login API, and you input your key to use the API:
from huggingface_hub import login
login(“”)
You’ll get this message if you’ve loaded it correctly. If so, congratulations, you successfully called the login API:

Figure 3.4 – Successful login and initialization
Now comes the fun part. We are going to use the Hugging Face Transformer API to take a line of text and turn it into an image. But first, we must import a Hugging Face agent using the HfAgent API (see the pattern?):
from transformers import HfAgentagent = HfAgent(“https://api-inference.huggingface.co/models/ bigcode/starcoderbase”)
We are using the starcoderbase model for this. Once you run this and get the agent, you can simply type in a prompt to generate an image:
agent.run(“Draw me a picture of prompt
“, prompt=”rainbow butterflies”)
But remember, if you don’t want to wait half an hour for your image, use the GPU runtime by going to the runtime tab and selecting it:
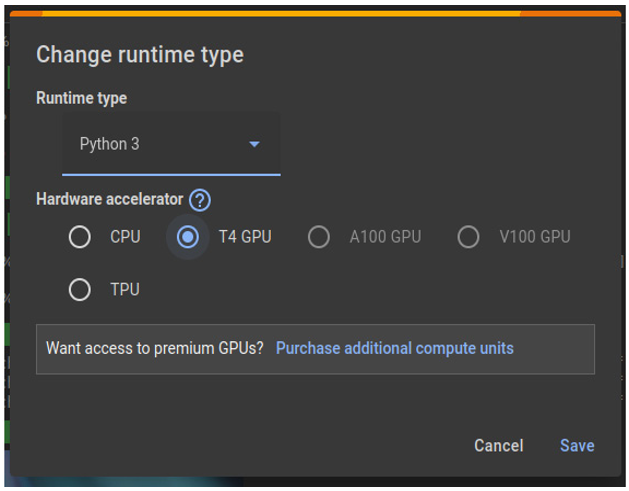
Figure 3.5 – Choosing a GPU for faster image processing
- The end product will leave you shocked and satisfied. You’ll get something like this:
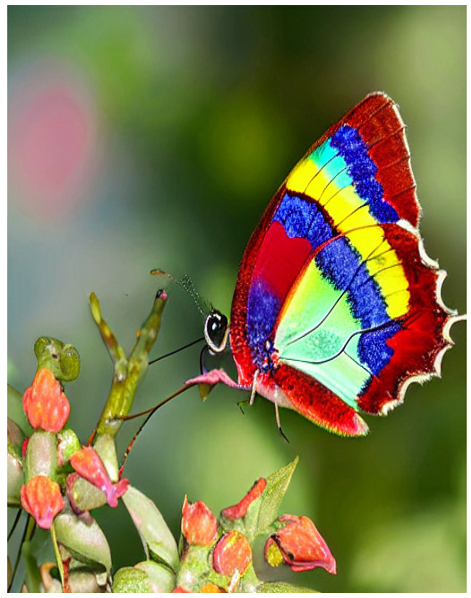
Figure 3.6 – Your final result (beautiful, isn’t it?)
So, we have completed this exercise and successfully called an API that has given us a visibly satisfying conclusion. What more could one ask for? Now, if only other people could witness the fruits of your labor!
Well, that’s what calling APIs is all about. APIs are meant to be consumed by your target audience and so now, we are going to see how we can distribute our APIs.